|
Michael Thomas Flanagan's Java Scientific Library
ImpedSpecSimulation Class: Impedance Spectroscopy Simulation
|
|
|
Last update: 5 July 2008
This class provides the methods for simulating complex impedance versus frequency spectra as used in impedance spectroscopy (IS) and in electrochemical impedance spectroscopy (EIS). The class allows for the simulation of:
- the complex impedance of the test or model circuit as a function of frequency
- the simulation of the voltage appearing across the test or model circuit when placed in the voltage divider:
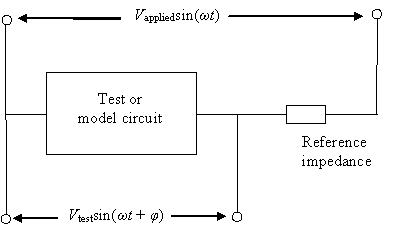
The inclusion of a reference impedance and an appied voltage is optional.
The users may provide their own test circuits, which may be implemented via an interface, ImpedSpecModel, or choose one of the 44 model circuits provided.
The circuit may contain any or all of the following electrical or electrochemical components:
- Resistor [complex impedance: R + j0]
- Capacitor  [complex impedance: 0 − j/Cω]
- Inductor [complex impedance: 0 + jLω]
- Constant Phase Element [complex impedance: σ(jω)−α]
- Warburg Impedance (infinite diffusion layer thickness) [complex impedance: σ/ω0.5 − jσ/ω0.5]
- Warburg Impedance (finite diffusion layer thickness) [complex impedance: σ/ω0.5(1 − j)tanh[δ(jω)0.5]]
The symbol, j, is used on this page to represent the square root of minus one.
import statement:
|
import flanagan.circuits.ImpedSpecSimulation;
|
Related classes
SUMMARY OF CONSTRUCTORS AND METHODS
Constructors
|
public ImpedSpecSimulation()
|
public ImpedSpecSimulation(String title)
|
Enter the circuit model
|
public void setModel(int modelNumber)
|
public void setModel(int modelNumber, double[ ] parameters)
|
public void setModel(int modelNumber, double[ ] parameters, String[ ] symbols)
|
public void setModel(ImpedSpecModel userModel, double[ ] parameters)
|
public void setModel(ImpedSpecModel userModel, double[ ] parameters, String[ ] symbols)
|
Enter the reference impedance
|
public void setReferenceImpedance(double resistance)
|
public void setReferenceImpedance(Complex referenceImpedance)
|
public void setReferenceImpedance(double refRealZ, double refImagZ)
|
Enter the applied voltage
|
public void setAppliedVoltage(double appliedVoltage)
|
Set the frequency range
|
public void setScanRangeHz(double low, double high)
|
public void setScanRangeRadians(double low, double high)
|
public void setLowFrequency(double low)
|
public void setLowRadialFrequency(double low)
|
public void setHighFrequency(double high)
|
public void setHighRadialFrequency(double high)
|
public void setLog10Plot()
|
public void setLinearPlot()
|
Return all calculated impedance and frequency data
|
public Vector<Object> getSimulationResults(int numberOfPoints)
|
Plot the impedance spectra
|
public void plotColeCole()
|
public void plotImpedanceMagnitudes()
|
public void plotImpedancePhases()
|
Plot the voltage spectra
|
public void plotVoltageMagnitudes()
|
public void plotVoltagePhases()
|
Write results to a file
|
public void printToTextFile(String fileName, int numberOfPoints)
|
public void printToTextFile(int numberOfPoints)
|
public void printToExcelFile(String fileName, int numberOfPoints)
|
public void printToExcelFile(int numberOfPoints)
|
The following methods in the class Impedance may be useful in building a user supplied circuit model where the user supplied class implementing the ImpedSpecModel interface may also extend Impedance.
Resistor impedance
|
public static Complex resistanceImpedance(double resistorValue)
|
Capacitor impedance
|
public static Complex capacitanceImpedance(double capacitorValue, double omega)
|
Inductor impedance
|
public static Complex inductanceImpedance(double inductorValue, double omega)
|
'Infinite' Warburg impedance
|
public static Complex infiniteWarburgImpedance(double sigma, double omega)
|
'Finite' Warburg impedance
|
public static Complex finiteWarburgImpedance(double sigma, double delta, double omega)
|
Constant phase element impedance
|
public static Complex constantPhaseElementImpedance(double sigma, double alpha, double omega)
|
Precompiled model circuit impedance
|
public static Complex
modelImpedance(double[] parameters, double omega, int modelNumber)
|
Impedances in series
|
public static Complex
impedanceInSeries(Complex impedance1, Complex impedance2)
|
public static Complex
impedanceInSeries(double impedance1, Complex impedance2)
|
public static Complex
impedanceInSeries(Complex impedance1, double impedance2)
|
public static Complex
impedanceInSeries(double impedance1, double impedance2)
|
public static Complex
rInSeriesWithC(double resistorValue, double capacitorValue, double omega)
|
public static Complex
rInSeriesWithL(double resistorValue, double inductorValue, double omega)
|
public static Complex
cInSeriesWithL(double capacitorValue, double inductorValue, double omega)
|
Impedances in parallel
|
public static Complex
impedanceInParallel(Complex impedance1, Complex impedance2)
|
public static Complex
impedanceInParallel(double impedance1, Complex impedance2)
|
public static Complex
impedanceInParallel(Complex impedance1, double impedance2)
|
public static Complex
impedanceInParallel(double impedance1, double impedance2)
|
public static Complex
rInParallelWithC(double resistorValue, double capacitorValue, double omega)
|
public static Complex
rInParallelWithL(double resistorValue, double inductorValue, double omega)
|
public static Complex
cInParallelWithL(double capacitorValue, double inductorValue, double omega)
|
List of precompiled model circuit components
|
public static String[ ]
modelComponents(int modelNumber)
|
Warburg coefficient, σ
|
public static double
warburgSigma(double electrodeArea, double oxidantDiffCoeff, double reductantDiffCoeff, double oxidantConcn, double reductantConcn, int electronsTransferred)
|
Parallel plate capacitance
|
public static double
parallelPlateCapacitance(double plateArea, double plateSeparation, double relativePermittivity)
|
public static double
parallelPlateCapacitance(double plateLength, double plateWidth, double plateSeparation, double relativePermittivity)
|
Coaxial cylinders - capacitance
|
public static double
coaxialCapacitance(double cylinderLength, double innerRadius, double outerRadius, double relativePermittivity)
|
Parallel wires - capacitance
|
public static double
parallelWiresCapacitance(double wireLength, double wireRadius, double wireSeparation, double relativePermittivity)
|
Parallel plates - inductance
|
public static double parallelPlateInductance(double plateLength, double plateWidth, double plateSeparation, double relativePermeability)
|
Coaxial cylinders - inductance
|
public static double
coaxialInductance(double cylinderLength, double innerRadius, double outerRadius, double relativePermbeability)
|
Parallel wires - inductance
|
public static double
parallelWiresInductance(double wireLength, double wireRadius, double wireSeparation, double relativePermbeability)
|
Magnitudes and phases
|
public static double
getMagnitude(Complex variable)
|
public static double
getPhaseRad(Complex variable)
|
public static double
getPhaseDeg(Complex variable)
|
Conversions
|
public static Complex
polarRad(double magnitude, double phaseRad)
|
public static Complex
polarDeg(double magnitude, double phaseDeg)
|
public static double frequencyToRadialFrequency(double frequency) |
public static double radialFrequencyToFrequency(double radial) |
CONSTRUCTORS
public ImpedSpecSimulation()
Usage:
ImpedSpecSimulation iss = new ImpedSpecSimulation();
Creates an instance of ImpedSpecSimulation for the simulation of an impedance spectrum.
public ImpedSpecSimulation(String title)
Usage:
ImpedSpecSimulation iss = new ImpedSpecSimulation(title);
Creates an instance of ImpedSpecSimulation for the simulation of an impedance spectrum. The argument title contains a title that will be displayed on plots and printed in the header of the output text file.
METHODS
ENTER THE CIRCUIT MODEL
A precompiled model circuit may be chosen or a user supplied model circuit may be entered
Choose one of the precompiled circuit models
public void setModel(int modelNumber, double[ ] parameters)
Usage:
iss.setModel(modelNumber, parameters);
This methods allows the selection of a precomplied model circuit. The available models are listed in ImpedSpecCircuitModels.pdf. The model circuits are listed in this pdf as:
- a model number (to be entered as the argument modelNumber);
- a list of the circuit parameter symbols as used in the input and output methods of this class;
- a diagram of the circuit
The values of the parameters should be entered, in SI units, via the array of doubles, argument parameters, and they should be in the same order as the list of parameter symbols referred to in (ii) above.
public void setModel(int modelNumber,double[ ] parameters, String[ ] symbols)
Usage:
iss.setModel(modelNumber, parameters, symbols);
This methods allows the selection of a precomplied model circuit. The available models are listed in ImpedSpecCircuitModels.pdf. The model circuits are listed in this pdf as:
- a model number (to be entered as the argument modelNumber);
- a list of the circuit parameter symbols as used in the input and output methods of this class;
- a diagram of the circuit
The values of the parameters should be entered, in SI units, via the array of doubles, argument parameters, and they should be in the same order as the list of parameter symbols referred to in (ii) above. The symbols listed in (ii) above will be replaced by those entered, in the same parameter order, via the array of Strings, argument symbols.
public void setModel(int modelNumber)
Usage:
iss.setModel(modelNumber);
This methods allows the selection of a precomplied model circuit. The available models are listed in ImpedSpecCircuitModels.pdf. The model circuits are listed in this pdf as:
- a model number (to be entered as the argument modelNumber);
- a list of the circuit parameter symbols as used in the input and output methods of this class;
- a diagram of the circuit
This method will request the values of the parameters, via a series of dialog boxes, in the same order as the parameter symbols listed in (ii) above.
Enter a user supplied circuit model
public void setModel(ImpedSpecModel userModel, double[ ] parameters, String[ ] symbols)
Usage:
iss.setModel(userModel, parameters, symbols);
This method allows the user to enter their own circuit model, using the interface ImpedSpecModel, via the argument userModel [See Coding a user supplied circuit model]. The values of the parameters should be entered, in SI units, via the array of doubles, argument parameters, in the order detrmined by the coding in userModel. The symbols, to be used in the output text file, for the model circuit parameters are entered via the array of Strings, argument symbols. They must be in the same order as that of the parameter values in parameters.
public void setModel(ImpedSpecModel userModel, double[ ] parameters)
Usage:
iss.setModel(userModel, parameters);
This method allows the user to enter their own circuit model, using the interface ImpedSpecModel, via the argument userModel [See Coding a user supplied circuit model]. The values of the parameters should be entered, in SI units, via the array of doubles, argument parameters, in the order detrmined by the coding in userModel. The parameters are simply labelled P1, P2, P3, ... etc. in the output text file.
Coding a user supplied circuit model
A class that extends the superclass Impedance and implements the interface ImpedSpecModel should be created. This class should contain a method named modelImpedance with the signature:
public Complex modelImpedance(double[ ] parameters, double omega)
This method should calculate and return the complex impedance of the user supplied circuit using the circuit parameter values passed through the argument, parameters, at the radial frequency passed through the argument, omega. The methods for calculating impedances, inherited from the superclass, Impedance and listed above, may be used in this method. A program demonstrating the use of a user supplied circuit is included in the Example Programs (Example Program Two).
SET THE REFERENCE IMPEDANCE
The reference impedance may be entered as a resistance or as a complex impedance. If it is not entered this class's calculation methods will calculate the test circuit impedances only and not the test circuit voltages.
public void setReferenceImpedance(double resistance)
Usage:
iss.setReferenceImpedance(resistance);
Sets the reference impedance to the resistance value, resistance.
public void setReferenceImpedance(double refRealZ, double refImagZ)
Usage:
iss.setReferenceImpedance(realZ, imagZ);
Sets the reference impedance to the complex impedance whose real part is realZ and whose real part is imagZ.
public void setReferenceImpedance(Complex referenceImpedance)
Usage:
iss.setReferenceImpedance(referenceImpedance);
Sets the reference impedance to the complex impedance referenceImpedance.
SET THE APPLIED VOLTAGE
public void setAppliedVoltage(double appliedVoltage)
Usage:
iss.setAppliedVoltage(appliedVoltage);
Sets the applied voltage to the value appliedVoltage.
If an applied voltage is not entered this class's calculation methods will calculate the test circuit impedances only and not the test circuit voltages.
SET THE FREQUENCY RANGE
The impedance spectra are calculated for 800 points between a given low frequency and high frequency. The points are calculated on a log to base 10 scale but this default option may be changed to a linear scale. Frequencies may be entered in Hz or as radial frequencies.
Set the high and low frequency points
public void setScanRangeHz(double low, double high)
Usage:
iss.setScanRangeHz(low, high);
The low and high frequencies bounding, inclusively, the scan range may be entered, in Hz, through this method's arguments, low and high.
public void setScanRangeRadians(double low, double high)
Usage:
iss.setScanRangeRadians(low, high);
The low and high radial frequencies bounding, inclusively, the scan range may be entered through this method's arguments, low and high.
public void setLowFrequency(double low)
Usage:
iss.setLowFrequency(low);
The lower frequency, setting inclusively the lowest point of the scan range, may be entered, in Hz, through this method's argument, low.
public void setLowRadialFrequency(double low)
Usage:
iss.setLowRadialFrequency(low);
The lower radial frequency, setting inclusively the lowest point of the scan range, may be entered through this method's argument, low.
public void setHighFrequency(double high)
Usage:
iss.setHighFrequency(high);
The higher frequency, setting inclusively the highest point of the scan range, may be entered, in Hz, through this method's argument, high.
public void setHighRadialFrequency(double high)
Usage:
iss.setHighRadialFrequency(high);
The higher radial frequency, setting inclusively the highest point of the scan range, may be entered through this method's argument, high.
Reset the log/linear scale option
public void setLinearPlot()
Usage:
iss.setLinearPlot();
This method resets the calculation option from arranging the 800 points in the frequency range from being equally spaced on a log to the base 10 scale to arranging them as equally spaced on a linear scale.
public void setLog10Plot()
Usage:
iss.setLog10Plot();
This method resets the calculation option from arranging the 800 points in the frequency range from being equally spaced on a linear to arranging them as equally spaced on a log to the base 10 scale. The log scale is the default option and this method only needs calling if the default option needs resetting after a change to a linear scale has been made (see immediately above)
RETURN ALL CALCULATED IMPEDANCE AND FREQUENCY DATA
public Vector<Object> getSimulationResults(int numberOfPoints)
Usage:
Vector<Object> alldata = iss.getSimulationResults(numberOfPoints);
This method calculates the impedance spectrum and returns a Vector whose elements contain:
- element 0: The circuit's complex impedences as an array of Complex
- element 1: The real part of the complex impedences as an array of doubles
- element 2: The imaginary part of the complex impedences as an array of doubles
- element 3: The magnitudes of the circuit's impedences as an array of doubles
- element 4: The phases of the circuit's impedences, in degrees, as an array of doubles
- element 5: The phases of the circuit's impedences, in radians, as an array of doubles
- element 6: The frequencies used, in Hz, as an array of doubles
- element 7: The log10[frequencies/Hz] used as an array of doubles
- element 8: The radial frequencies used as an array of doubles
and if the applied voltage and reference impedance have been entered
- element 9: The applied voltage as a Double
- element 10: The reference impedance as a Complex
- element 11: The complex voltages appearing across the test circuit as an array of Complex
- element 12: The real parts of the voltages appearing across the test circuit as an array of doubles
- element 13: The imaginary parts of the voltages appearing across the test circuit as an array of doubles
- element 14: The magnitudes of the voltages appearing across the test circuit as an array of doubles
- element 15: The phases, in degrees, of the voltages appearing across the test circuit as an array of doubles
- element 16: The phases, in radians, of the voltages appearing across the test circuit as an array of doubles
for numberOfPoints equally spaced points between the lowest and highest frequency inclusively. If a numberOfPoints is entered as a integer greater than 800 it will be replaced by 800. The frequency scale will be a log to base 10 scale unless the method setLinearPlot() had been called.
PLOT THE IMPEDANCE SPECTRA
public void plotColeCole()
Usage:
iss.plotColeCole();
Displays a Cole-Cole plot, i.e. a plot of the imaginary parts of the complex test circuit impedance against the real parts of the complex impedance.
public void plotImpedanceMagnitudes()
Usage:
iss.plotImpedanceMagnitudes();
Displays a plot of the magnitudes of the test circuit impedance against the log10 of the frequency (Hz) or, if the method setLinearPlot() has been set, against the frequency in Hz.
public void plotImpedancePhases()
Usage:
iss.plotImpedancePhases();
Displays a plot of the phases of the circuit impedance, in degrees, against the log10 of the frequency (Hz) or, if the method setLinearPlot() has been set, against the frequency in Hz.
PLOT THE VOLTAGE SPECTRA
public void plotVoltageMagnitudes()
Usage:
iss.plotVoltageMagnitudes();
Displays a plot of the magnitudes of the voltages appearing across the test circuit against the log10 of the frequency (Hz) or, if the method setLinearPlot() has been set, against the frequency in Hz.
public void plotVoltagePhases()
Usage:
iss.plotVoltagePhases();
Displays a plot of the phases of the voltages appearing across the test circuit, in degrees, against the log10 of the frequency (Hz) or, if the method setLinearPlot() has been set, against the frequency in Hz.
WRITE RESULTS TO A FILE
public void printToTextFile(String fileName, int numberOfPoints)
Usage:
iss.printToTextFile(fileName, numberOfPoints);
Prints to a text file, named fileName, the following:
- program title
- file name
- time and date that the file was created
- circuit parameters and their values
- frequencies in Hz
- real parts of the circuit impedances
- imaginary parts of the circuit impedances
- magnitudes of the circuit impedances
- phases, in degrees, of the circuit impedances
- phases, in radians, of the circuit impedances
- log10[frequencies/Hz]
- radial frequencies
and, if an applied voltage and a reference impedance have been entered,
- applied voltage
- reference impedance
- frequencies in Hz
- real parts of the test circuit voltages
- imaginary parts of test circuit voltages
- magnitudes of the test circuit voltages
- phases, in degrees, of the test circuit voltages
- phases, in radians, of the test circuit voltages
- log10[frequencies/Hz]
- radial frequencies
for numberOfPoints equally spaced points between the lowest and highest frequency inclusively. If a numberOfPoints is entered as a integer greater than 800 it will be replaced by 800. The frequency scale will be a log to base 10 scale unless the method setLinearPlot() had been called.
public void printToTextFile(int numberOfPoints)
Usage:
iss.printToTextFile(numberOfPoints);
Prints to a text file, named ImpedSpecSimulationOutputn.txt, the data listed above for method print(fileName, numberOfPoints). The integer n in the file name starts at 1 and is incremented by 1 every time the method is called without the previous output file being removed from the working directory.
public void printToExcelFile(String fileName, int numberOfPoints)
Usage:
iss.printToExcelFile(fileName, numberOfPoints);
Prints to a file, named fileName.xls, the data listed above for method print(fileName, numberOfPoints). This file is in a format that facilitates the display of the data columns as Excel sheet columns when this file is opened with Microsoft Excel.
public void printToExcelFile(int numberOfPoints)
Usage:
iss.printToExcelFile(numberOfPoints);
Prints to a file, named ImpedSpecSimulationOutputn.xls, the data listed above for method print(fileName, numberOfPoints). This file is in a format that facilitates the display of the data columns as Excel sheet columns when this file is opened with Microsoft Excel. The integer n in the file name starts at 1 and is incremented by 1 every time the method is called without the previous output file being removed from the working directory. This file can be read by MS Excel maintaining the data columns as Excel sheet columns.
METHODS IN THE CLASS, Impedance
These may be useful in building a user supplied circuit model. The user supplied class implementing the interface ImpedSpecModel may also extend Impedance.
Resistor impedance
|
public static Complex resistanceImpedance(double resistorValue)
Usage:
imped = Impedance.resistanceImpedance(resistorValue);
Returns the impedance, Z, of a resistor, R [resitorValue entered in ohms], as a complex impedance:
Z = R + j0
Capacitor impedance
public static Complex capacitanceImpedance(double capacitorValue, double omega)
Usage:
imped = Impedance.capacitanceImpedance(capacitorValue, omega);
Returns the complex impedance, Z, of a capacitor, C [CapacitorValue entered in farads] at a radial frequency ω [omega]:
Z = 0 − j/Cω
Inductor impedance
public static Complex inductanceImpedance(double inductorValue, double omega)
Usage:
imped = Impedance.inductanceImpedance(inductorValue, omega);
Returns the complex impedance, Z, of a inductor, L [inductorValue entered in henries] at a radial frequency ω [omega]:
Z = 0 + jLω
'Infinite' Warburg impedance
public static Complex infiniteWarburgImpedance(double sigma, double omega)
Usage:
imped = Impedance.infiniteWarburgImpedance(sigma, omega);
Returns the Warburg impedance, Z, for an infinite diffusion layer and a constant, σ [sigma], at a radial frequency ω [omega]:
Z = σ/ω0.5 − jσ/ω0.5
See also Warburg coefficient, σ.
'Finite' Warburg impedance
public static Complex finiteWarburgImpedance(double sigma, double delta, double omega)
Usage:
imped = Impedance.finiteWarburgImpedance(sigma, delta, omega);
Returns the Warburg impedance, Z, for a finite diffusion layer and constants, σ [sigma] and δ [delta], at a radial frequency ω [omega]:
Z = σ/ω0.5(1 − j)tanh[δ(jω)0.5]
The parameter, δ, equals d/D-0.5 where d is the diffusion layer thickness and D is the relevant diffusion coefficient. The full finite Warburg impedance is the series combination:
Zfull = σo/ω0.5(1 − j)tanh[δo(jω)0.5] + σr/ω0.5(1 − j)tanh[δr(jω)0.5]
where the subscripts refer to the oxidant and reductant species respectively.
Constant phase element impedance
public static Complex constantPhaseElementImpedance(double sigma, double alpha, double omega)
Usage:
imped = Impedance.constantPhaseElementImpedance(sigma, alpha, omega);
Returns the impedance, Z, of a Constant Phase Element with constants, σ [sigma] and α [alpha], at a radial frequency ω [omega]:
Z = σ(jω)−α
Precompiled model circuit impedance
public static Complex
modelImpedance(double[] parameters, double omega, int modelNumber)
Usage:
imped = Impedance.modelImpedance(sigma, alpha, omega);
Returns the complex impedance of a designated model chosen from the list of precompiled circuit models at a radial frequency ω [omega]. The model number is entered as the argument, modelNumber, and the circuit parameters must be entered through the argument parameters in the same order as the list of parameter symbols to be found after the model number in ImpedSpecCircuitModels.pdf
Impedances in series
|
public static Complex
impedanceInSeries(Complex impedance1, Complex impedance2)
public static Complex
impedanceInSeries(double impedance1, Complex impedance2)
public static Complex
impedanceInSeries(Complex impedance1, double impedance2)
public static Complex
impedanceInSeries(double impedance1, double impedance2)
Usage:
imped = Impedance.impedanceInSeries(impedance1, impedance1);
Returns the complex impedance of a two impedances [arguments impedance1 and impedance2] in series. The impedances impedance1 and impedance2 may be complex or real (resistance).
public static Complex
rInSeriesWithC(double resistorValue, double capacitorValue, double omega)
Usage:
imped = Impedance.rInSeriesWithC(resistorValue, capacitorValue, omega);
Returns the complex impedance, at a radial frequency ω [argument omega], of a resistor [argument resistorValue (ohms)] in series with a capacitor [argument resistorValue (farads)].
public static Complex
rInSeriesWithL(double resistorValue, double inductorValue, double omega)
Usage:
imped = Impedance.rInSeriesWithL(resistorValue, inductorValue, omega);
Returns the complex impedance, at a radial frequency ω [argument omega], of a resistor [argument resistorValue (ohms)] in series with an inductor [argument inductorValue (henries)].
public static Complex
cInSeriesWithL(double capacitorValue, double inductorValue, double omega)
Usage:
imped = Impedance.cInSeriesWithL(capacitorValue, inductorValue, omega);
Returns the complex impedance, at a radial frequency ω [argument omega], of a capacitor [argument capacitorValue (farads)] in series with an inductor [argument inductorValue (henries)].
Impedances in parallel
|
public static Complex
impedanceInParallel(Complex impedance1, Complex impedance2)
public static Complex
impedanceInParallel(double impedance1, Complex impedance2)
public static Complex
impedanceInParallel(Complex impedance1, double impedance2)
public static Complex
impedanceInParallel(double impedance1, double impedance2)
Usage:
imped = Impedance.impedanceInParallel(impedance1, impedance1);
Returns the complex impedance of a two impedances [arguments impedance1 and impedance2] in parallel. The impedances impedance1 and impedance2 may be complex or real (resistance).
public static Complex
rInParallelWithC(double resistorValue, double capacitorValue, double omega)
Usage:
imped = Impedance.rInParallelWithC(resistorValue, capacitorValue, omega);
Returns the complex impedance, at a radial frequency ω [argument omega], of a resistor [argument resistorValue (ohms)] in parallel with an capacitor [argument capacitorValue (farads)].
public static Complex
rInParallelWithL(double resistorValue, double inductorValue, double omega)
Usage:
imped = Impedance.rInParallelWithL(resistorValue, inductorValue, omega);
Returns the complex impedance, at a radial frequency ω [argument omega], of a resistor [argument resistorValue (ohms)] in parallel with an inductor [argument inductorValue (henries)].
public static Complex
cInParallelWithL(double capacitorValue, double inductorValue, double omega)
Usage:
imped = Impedance.cInParallelWithL(capacitorValue, inductorValue, omega);
Returns the complex impedance, at a radial frequency ω [argument omega], of a capacitor [argument capacitorValue (farads)] in parallel with an inductor [argument inductorValue (henries)].
List of precompiled model circuit components
|
public static String[ ] modelComponents(int modelNumber)
Usage:
symbols = Impedance.modelComponents(modelNumber);
Returns an array of the parameter symbols, as listed in ImpedSpecCircuitModels.pdf, for a circuit selected from the range of precompiled circuit models. The model number is entered via the argument modelNumber.
Warburg Coefficient, σ
public static double
warburgSigma(double electrodeArea, double oxidantDiffCoeff, double reductantDiffCoeff, double oxidantConcn, double reductantConcn, int electronsTransferred)
Usage:
cap = Impedance.warburgSigma(electrodeArea, oxidantDiffCoeff, reductantDiffCoeff, oxidantConcn, reductantConcn, electronsTransferred);
Returns the warburg coefficient, σ,
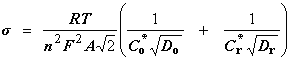
of elecrtode area, A square metres, [argument electrodeArea], oxidant diffusion coefficient, Do square metres per second, [argument oxidantDiffCoeff], reductant diffusion coefficient, Dr square metres per second, [argument reductantDiffCoeff], oxidant concentration at the electrode surface, C*o moles per cubic metre, [argument oxidantConcn], reductant concentration at the electrode surface, C*r moles per cubic metre, [argument reductantConcn] and the number of electrons transferred in the electrochemical reaction,
nr, [argument electronsTransferred].
Parallel Plate Capacitance
The plate area may be entered as an area or, additionally for rectangular plates, as a length and a width.
public static double parallelPlateCapacitance(double plateArea, double plateSeparation, double relativePermittivity)
Usage:
cap = Impedance.parallelPlateCapacitance(plateArea, plateSeparation, relativePermittivity);
Returns the capacitance, C in farads,
C = Aεoεr/d
of a parallel plate capacitor of plate area, A square metres, [argument plateArea], plate separation d metres, [argument plateArea] and dielectric relative electrical permittivity,
εr [argument relativePermittivity]. See classes
Water for the relative permittivity of water. Plate edge effects are ignored.
public static double parallelPlateCapacitance(double plateLength, double plateWidth, double plateSeparation, double relativePermittivity)
Usage:
cap = Impedance.parallelPlateCapacitance(plateLength, plateWidth, plateSeparation, relativePermittivity);
Returns the capacitance, C in farads,
C = lwεoεr/d
of a parallel plate capacitor of plate length, l metres, [argument plateLength], plate width, w metres, [argument plateWidth], plate separation d metres, [argument plateArea] and dielectric relative electrical permittivity,
εr [argument relativePermittivity]. See classes
Water for the relative permittivity of water. Plate edge effects are ignored.
Coaxial Cylinders - Capacitance
public static double
coaxialCapacitance(double cylinderLength, double innerRadius, double outerRadius, double relativePermittivity)
Usage:
cap = Impedance.coaxialCapacitance(cylinderLength, innerRadius, outerRadius, relativePermittivity);
Returns the capacitance, C in farads, of two coaxial cylinders,
C = 2lπεoεr/ln(router/router)
where l is the length of the cylinders in metres [argument cylinderLength], rinner is the radius from the coaxial centre point to the outer surface of the inner cylinder in metres [argument innerRadius], router is the radius from the coaxial centre point to the inner surface of the outer cylinder in metres[argument outerRadius] and εr is relative electrical permittivity of the dielectric between the inner and outer cylinders
[argument relativePermittivity]. Cylinder end effects are ignored.
Parallel Wires - Capacitance
public static double
parallelWiresCapacitance(double wireLength, double wireRadius, double wireSeparation, double relativePermittivity)
Usage:
cap = Impedance.parallelWiresCapacitance(wireLength, wireRadius, wireSeparation, relativePermittivity);
Returns the capacitance, C in farads, of two parallel wires,
C = lπεoεr/ln((d - r)/r)
where l is the length of the wires in metres [argument wireLength], r is the radius of the wires in metres [argument wiresRadius], d is the separation distance of the wires, measured from the centres of the wires, in metres [argument wireSeparation] and εr is relative electrical permittivity of the dielectric surrounding the wires [argument relativePermittivity]. It is assumed that the wires have identical radii and that the this radius is much smaller than the distance between the wires (no polarisation effects are considered) and wire end effects are ignored.
Parallel Plates - Inductance
public static double parallelPlateInductance(double plateLength, double plateWidth, double plateSeparation, double relativePermeability)
Usage:
ind = Impedance.parallelPlateInductance(plateLength, plateWidth, plateSeparation, relativePermeability);
Returns the inductance, L in henries,
C = ldμoμr/w
of a parallel plates of plate length, l metres, [argument plateLength], plate width, w metres, [argument plateWidth], plate separation d metres, [argument plateArea] and μr is relative magnetic permeability of the material between the plates
[argument relativePermeability]. Plate edge effects are ignored.
Coaxial Cylinders - Inductance
public static double
coaxialInductance(double cylinderLength, double innerRadius, double outerRadius, double relativePermeability)
Usage:
ind = Impedance.coaxialInductance(cylinderLength, innerRadius, outerRadius, relativePermeability);
Returns the capacitance, L in henrys, of two coaxial cylinders,
L = lμoμr
ln(router/router)/(2π)
where l is the length of the cylinders in metres [argument cylinderLength], rinner is the radius from the coaxial centre point to the outer surface of the inner cylinder in metres [argument innerRadius], router is the radius from the coaxial centre point to the inner surface of the outer cylinder in metres[argument outerRadius] and μr is relative magnetic permeability of the material between the inner and outer cylinders
[argument relativePermeability]. Cylinder end effects are ignored.
Parallel Wires - Inductance
public static double
parallelWiresInductance(double wireLength, double wireRadius, double wireSeparation, double relativePermeability)
Usage:
ind = Impedance.parallelWiresInductance(wireLength, wireRadius, wireSeparation, relativePermeability);
Returns the capacitance, L in henrys, of two parallel wires,
L = lμoμrln((d - r)/r)/π
where l is the length of the wires in metres [argument wireLength], r is the radius of the wires in metres [argument wiresRadius], d is the separation distance of the wires, measured from the centres of the wires, in metres [argument wireSeparation] and μr is relative magnetic permeability of the material surrounding the wires [argument relativePermeability]. It is assumed that the wires have identical radii and that the this radius is much smaller than the distance between the wires (no polarisation effects are considered) and wire end effects are ignored.
Magnitudes and phases
public static double
getMagnitude(Complex variable)
Usage:
mag = Impedance.getMagnitude(complexVariable);
Returns the modulus, i.e. the magnitude, of a complex variable [complexVariable].
public static double
getPhaseRad(Complex variable)
Usage:
phaseRadians = Impedance.getPhaseRad(complexVariable);
Returns the argument, i.e. the phase, in radians, of a complex variable [complexVariable].
public static double
getPhaseDeg(Complex variable)
Usage:
phaseDegrees = Impedance.getPhaseDeg(complexVariable);
Returns the argument, i.e. the phase, in degrees, of a complex variable [complexVariable].
Conversions
public static Complex
polarRad(double magnitude, double phaseRad)
Usage:
complexVariable = Impedance.polarRad(magnitude, phaseRadians);
Converts the phasor of magnitude, magnitude, and phase in radians, phaseRadians, to the rectangular complex variable, complexVariable,
magnitude.cos(phaseRadians) + j.magnitude.sin(phaseRadians).
public static Complex
polarDeg(double magnitude, double phaseDeg)
Usage:
complexVariable = Impedance.polarDeg(magnitude, phaseDegrees);
Converts the phasor of magnitude, magnitude, and phase in degrees, phaseDegrees, to the rectangular complex variable, complexVariable,
magnitude.cos(2.π.phaseDegrees/180) + j.magnitude.sin(2.π.phaseDegrees/180).
Frequency (Hz) to radial frequency
public static double frequencyToRadialFrequency(double freq) Usage:
radial =
Fmath.frequencyToRadialFrequency(freq); Converts the argument, freq, a frequency in Hz, to
the corresponding radial frequency and returns that value in radians per second.
Radial frequency to frequency
public static double radialfrequencyToFrequency(double rad)
Usage:
freq =
Fmath.radialFrequencyToFrequency(rad); Converts the argument, rad, a radial frequency in radians per second, to
the corresponding frequency and returns that value in Hz.
EXAMPLE PROGRAMS
Example Program One
This program demonstrates the choice of a model incorporating a constant phase element (Q1), model number 26,
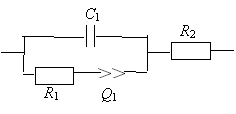
from the range of precompliled circuit models.
Example Program One source code: ExampleProgramOneISS.java
Example Program Two
This program demonstrates the use of the interface ImpedSpecModel with a user supplied model circuit
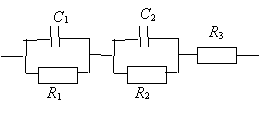
Example Program Two source code: ExampleProgramTwoISS.java
OTHER CLASSES USED BY THIS CLASS
This class uses the following classes in this library:
This page was prepared by Dr Michael Thomas Flanagan
|